JWT (JSON Web Token) is an open source standard commonly used to transmit data between two services in a compact and secure way. The information transmitted between services can be verified and trusted because it is digitally signed.
JWT Token is used to –
- Transfer identity between different entities.
- Transfer user entitlements between interested parties.
- Transfer data securely between interested parties over an unsecured channel.
- Assert other parties identity based on the information and authenticate to access the resources.
The signed JWT is a compact URL-safe string consist of three parts separated by periods (.) Ex: A.B.C here is A, B, C refer to the JOSE Header, the JWS Payload, and the JWS signature
JOSE Header . JWS Payload . JWS Signature
JSON Web Token (JWT) Structure
JOSE Header
- Algorithm – The hashing algorithm used
- Ex: RS256, HS256, HS384, HS512, RS384, PS384, ES256 etc.
- Type – Type of the token.
- Ex: JWT
- Certificate chain
- Ex: x5c –> X.509 Certificate Chain (Public Cert)
JWS Payload
- iss — Issuer
- sub — Subject
- aud — Audience
- exp — Expiration Time
- iat — Issued At
- so on…
JWS Signature
- The encoded header
- The encoded payload
- A secret
- An algorithm and sign
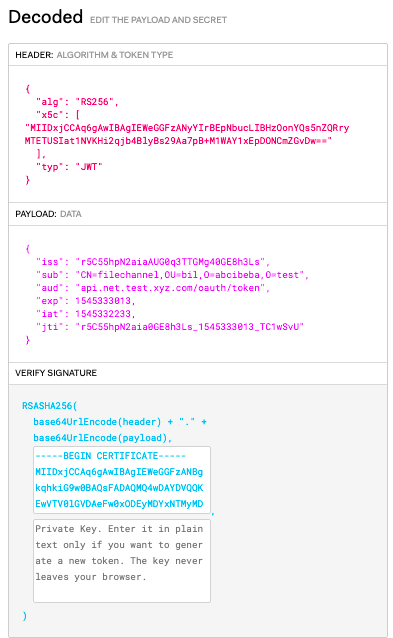
Create JWT Token
Installing
pip install PyJWT
Implementation
import jwt
import datetime as dt
from datetime import datetime
def generate_jwt_token(iss, sub, aud, x5c, signature, exptime):
now = dt.datetime.now()
current_time = int(datetime.timestamp(now))
expired_time = int(datetime.timestamp(now + dt.timedelta(minutes = exptime)))
payload = {
"iss":iss,
"sub":sub,
"aud":aud,
"exp":expired_time,
"iat":current_time
}
headers={
"alg":"RS256",
"x5c":x5c,
"typ":"JWT"
}
signature=signature
token = jwt.encode(payload, headers=headers, key=signature, algorithm="RS256")
token = token.decode("utf-8")
return token
In the example above –
The payload section is having an issuer, subject, audience, expiration time, issued time, and you can add more based on your requirement.
The header section is having an algorithm, x5c header (public key), and type as JWT.
Using JWT library encoding all three components payload, header, and signature.
To view the generated token use the token.decode(“utf-8”).