Karate is an open source library used to test the web services based on plain English in the text file called feature file. Karate is built on top of Cucumber, so it shares some of the concepts of descriptive language based on the Cucumber and it allows tests to be easily written and understood even by the non-programmers.
Karate is the only open-source tool to combine API test-automation, mocks and performance-testing into a single, unified framework. The BDD syntax popularized by Cucumber is language-neutral and easy for even non-programmers. Besides powerful JSON & XML assertions, you can run tests in parallel for speed – which is critical for HTTP API testing.
source: https://github.com/intuit/karate
Setup:
Maven
Just addkarate-apache
dependency to the pom.xml
and karate-junit4
dependency to facilitate JUnit testing:
You can use several of the testing frameworks – JUnit4, JUnit5, TestNG etc.
<dependencies>
<dependency>
<groupId>com.intuit.karate</groupId>
<artifactId>karate-apache</artifactId>
<version>0.9.2</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>com.intuit.karate</groupId>
<artifactId>karate-junit4</artifactId>
<version>0.9.2</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<testResources>
<testResource>
<directory>src/test/java</directory>
<excludes>
<exclude>**/*.java</exclude>
</excludes>
</testResource>
</testResources>
</build>
JUnit Runner
Create JUnit Test Runner file. A Simple JUnit Class will help to run the tests with the JUnit framework.
import org.junit.runner.RunWith;
import com.intuit.karate.junit4.Karate;
@RunWith(Karate.class)
public class TestRunner {
}
Karate Scenarios
Create a feature file and add your scenarios to it.
In the example below, I have created two scenarios one for GET and one for POST. I know this is very basic and simple test, however you can create complex requests and write matchers to compare the results.
Feature: ReqRes API to be tested
Background:
* url 'https://reqres.in'
* header Accept = 'application/json'
Scenario: Get a user
Given path '/api/users/2'
When method get
Then status 200
And match response.data.first_name == 'Janet'
Scenario: Post a user
Given path '/api/users'
And request { "name": "David", "job": "IT Consultant"}
When method post
Then status 201
And def user = response
And match $ contains {name: '#(user.name)', job: '#(user.job)'}
Parallel Execution
Karate can run tests in parallel and dramatically cut down execution time. This is a ‘core’ feature and does not depend on JUnit, Maven or TestNG.
a simple parallel execution file looks like this. –
import com.intuit.karate.KarateOptions;
import com.intuit.karate.Results;
import com.intuit.karate.Runner;
import static org.junit.Assert.*;
import org.junit.Test;
@KarateOptions(tags = {"~@ignore"})
public class TestParallel {
@Test
public void testParallel() {
Results results = Runner.parallel(getClass(), 6, "target/surefire-reports");
assertTrue(results.getErrorMessages(), results.getFailCount() == 0);
}
}
Parallel execution results:
--------------------------------------------------------- feature: classpath:com/karate/test/reqres.feature report: target/surefire-reports/com.karate.test.reqres.json scenarios: 2 | passed: 2 | failed: 0 | time: 1.7946 --------------------------------------------------------- --------------------------------------------------------- feature: classpath:com/karate/test/reqres2.feature report: target/surefire-reports/com.karate.test.reqres2.json scenarios: 2 | passed: 2 | failed: 0 | time: 1.7872 --------------------------------------------------------- Karate version: 0.9.2 ====================================================== elapsed: 1.40 | threads: 4 | thread time: 3.58 features: 2 | ignored: 0 | efficiency: 0.64 scenarios: 4 | passed: 4 | failed: 0 ======================================================
Test Execution Reports:
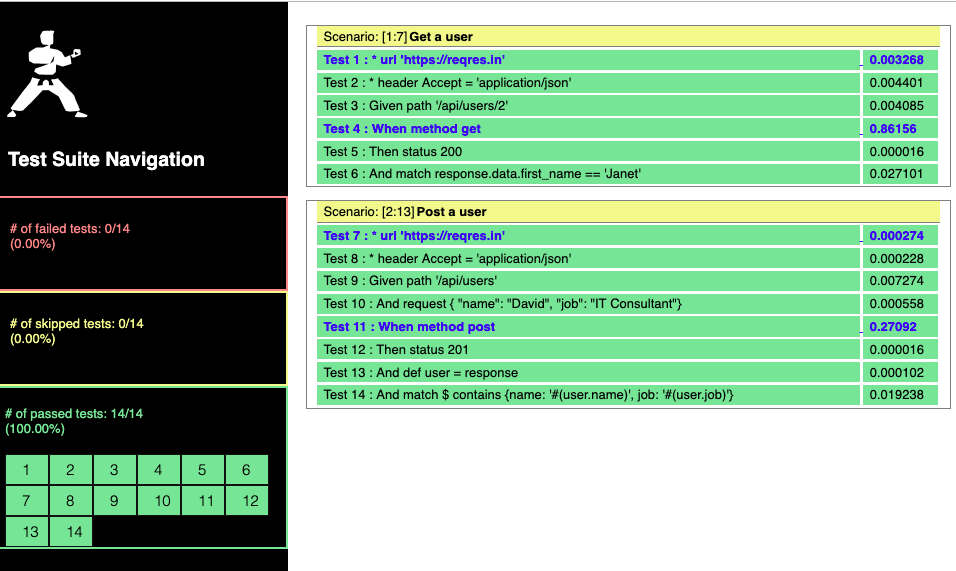
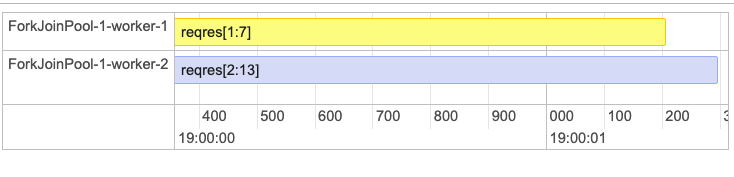
Conclusion
Karate comes with a very rich set of useful features which enables you to write Automated API scripts simple and easy to understand by non-programmers. Let’s see whether the Karate framework takes the API testing to the next level or not.